I created a PPT online and added a background image. After downloading it locally, I tried using Apache POI to convert it into an image. However, there was an error when converting it (if there is no background image, it can be converted normally).
Create a PPT locally using MS Office and use the same image as the background. It can be converted to an image using POI .
How can the background image added online be converted into an image normally?
error info:
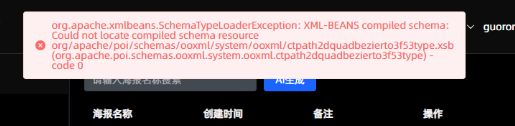
Hello @xuxiaofei,
Please provide the actual code that you use to convert the presentation file into images using Apache POI so we could test
MAVEN:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-scratchpad</artifactId>
<version>5.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>ooxml-schemas</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.29</version>
</dependency>
Code:
try {
String profile = "C:\\Users\\xuyongjian\\Desktop\\xpiory";
String pptUrl = "file:///" + "C:\\Users\\xuyongjian\\Desktop\\替换背景图.pptx";
System.out.println(pptUrl);
// 从 URL 下载 PPT 文件到临时目录
URL url = new URL(pptUrl);
InputStream inputStream = url.openStream();
// 判断是 PPT(.ppt)还是 PPTX(.pptx)格式
boolean isPPTX = pptUrl.toLowerCase().endsWith(".pptx");
if (isPPTX) {
XMLSlideShow ppt = new XMLSlideShow(inputStream);
// 遍历幻灯片并转换
Dimension slideSize = ppt.getPageSize();
for (XSLFSlide slide : ppt.getSlides()) {
System.out.println("开始转发pdf");
PDDocument pdfDoc = new PDDocument();
// 渲染幻灯片为 BufferedImage
BufferedImage image = new BufferedImage(slideSize.width, slideSize.height, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics = image.createGraphics();
slide.draw(graphics);
graphics.dispose();
// 添加 PDF 页面
PDPage pdfPage = new PDPage(new PDRectangle(slideSize.width, slideSize.height));
pdfDoc.addPage(pdfPage);
String pdf = profile + "/下载图片/" + System.currentTimeMillis() + ".pdf";
// 插入图片到 PDF
PDImageXObject pdImage = PDImageXObject.createFromByteArray(
pdfDoc, imageToBytes(image), "slide"
);
try (PDPageContentStream contentStream = new PDPageContentStream(pdfDoc, pdfPage)) {
contentStream.drawImage(pdImage, 0, 0, slideSize.width, slideSize.height);
}
pdfDoc.save(pdf);
System.out.println("开始转换png");
PDFRenderer renderer = new PDFRenderer(pdfDoc);
for (int page = 0; page < pdfDoc.getNumberOfPages(); page++) {
BufferedImage pdfImage = renderer.renderImageWithDPI(page, 300); // 设置 DPI
String outputPath = profile + "/下载图片/" + System.currentTimeMillis() + ".png";
ImageIO.write(pdfImage, "PNG", new File(outputPath));
}
pdfDoc.close();
}
ppt.close();
} else {
throw new RuntimeException("暂不支持.ppt格式文件");
}
inputStream.close();
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException(e);
}
Which version of the Document Server is used? I tested on 8.3.3 version and the conversion via Java application went as expected, the background image is still there in .pdf, no errors, also the image was saved separately after the program execution (but together with the text of the slide)